“ 지연되는 프로젝트에 인력을 더 투입하면 오히려 더 늦어진다. ”
- Frederick Philips Brooks
Mythical Man-Month 저자
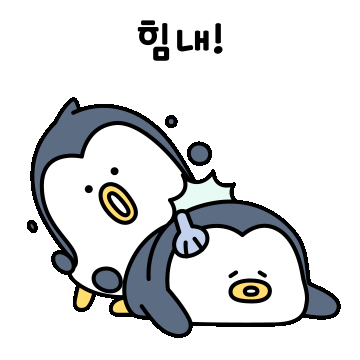
자바스크립트 데이터 저장은 크게 네 가지로 나눌 수 있습니다.
✍🏻 변수✍🏻
변수는 데이터를 저장하는 저장소입니다.
데이터를 저장할 수도 있지만 변경과 추가가 가능합니다.
데이터 변경이 가능하고, 마지막으로 지정해준 데이터가 값이 됩니다.
📍 데이터 저장
{
var x = 100;
var y = 200;
var z = "javascript";
console.log(x);
console.log(y);
console.log(z);
}
결과 :
100
200
javascript
📍 데이터 저장 + 변경
let x = 100;
let y = 200;
let z = "javascript";
x = 1000;
y = 1000;
z = "react";
document.write(x);
document.write(y);
document.write(z);
결과 :
1000
1000
react
📍 데이터 저장 + 변경 + 추가
{
let x = 200;
let y = 300;
let z = "javascript";
x += 300; //x = x + 300
y += 400;
z += "react";
console.log(x);
console.log(y);
console.log(z);
}
결과 :
500
700
javascriptreact
✍🏻 상수✍🏻
상수는 데이터 저장이 가능하지만, 변경이 불가능합니다.
상수는 이미 선언한 상수에 대해 중복 선언이 불가능하며 상수의 값을 재지정할 수도 없습니다.
객체는 중괄호({})를 사용하여 나타내며, 각 데이터와 동작은 속성(property)이라고 부릅니다.
객체의 속성은 이름과 값으로 이루어져 있으며 이름과 값 사이에는 (;)을 사용하여 구분합니다.
const x = 300;
const y = 100;
const z = "javascript";
x = 100;
y = 300;
z = "react";
document.write(x);
document.write(y);
document.write(z);
결과 :
출력 안 됨
📍 배열 1
배열은 데이터를 여러 개 저장할 수 있습니다.
const arr = new Array();
arr[0] = 500;
arr[1] = 1000;
arr[2] = "react";
document.write(arr[0]);
document.write(arr[1]);
document.write(arr[2]);
결과 :
5001000react
📍 배열 2
new Array()에 데이터를 ","로 구분하여 저장하는 방법
const arr = new Array(200, 600, "javascript");
document.write(arr[0]);
document.write(arr[1]);
document.write(arr[2]);
결과 :
200600javascript
📍 배열 3
new Array를 생략하고 인덱스에 데이터를 저장하는 방법
const arr = [];
arr [0] = 400;
arr [1] = 3300;
arr [2] = "javascript";
document.write(arr[0]);
document.write(arr[1]);
document.write(arr[2]);
결과 :
4003300javascript
📍 배열 4
new Array를 생략하고 대괄호 안에 데이터를 ","로 구분하여 저장하는 방법
const arr = [1020, 2020, "javascript"];
document.write(arr[0]);
document.write(arr[1]);
document.write(arr[2]);
결과 :
10202020javascript
📍 객체 1
객체는 데이터 값을 필요한대로 만들어 사용할 수 있습니다.
객체의 데이터는 키(이름): 값으로 이루어 있으며 이것을 속성이라고 합니다.
const obj = new Object();
obj[0] = 200;
obj[1] = 500;
obj[2] = "javascript";
document.write(obj[0]);
document.write(obj[1]);
document.write(obj[2]);
결과 :
200500javascript
📍 객체 2
숫자 대신 문자 a, b, c에 값을 직접 선언하는 방법
const obj = new Object();
obj.a = 100;
obj.b = 200;
obj.c = "자바java";
document.write(obj.a);
document.write(obj.b);
document.write(obj.c);
결과 :
100200java자바
📍 객체 3
new Object를 생략하고 중괄호를 써서 객체를 선언하는 방법
const obj = {};
obj.a = "안녕하세요.";
obj.b = "저는";
obj.c = "이유나입니다.";
document.write(obj.a);
document.write("<br>");
document.write(obj.b);
document.write("<br>");
document.write(obj.c);
결과 :
안녕하세요
저는
이유나입니다
📍 객체 4
객체 선언 코드를 생략하고 중괄호 안에 키와 값을 직접 입력해 저장하는 방법
const obj = {a:"열심히 한다면", b:" 반드시", c:" 멋진 결과가 !"};
document.write(obj.a);
document.write(obj.b);
document.write(obj.c);
결과 :
열심히 한다면 반드시 좋은 결과가 !
📍 객체 5
배열 안에 객체가 있는 구조는 밑의 방법으로 불러옵니다.
const arr = [
{a:"꾸준함은", b:" 어렵지만"},
{c: " 결과는 아름답다 !"}
];
document.write(arr[0].a);document.write(arr[0].b);document.write(arr[1].c);
결과 :
꾸준함은 어렵지만 결과는 아름답다 !
📍 객체 6
여러 개의 속성에서 지정된 값만 출력하려면 밑의 방법으로 불러옵니다.
const obj = {
a: 100,
b: [300,400],
c: {x: 500, y: 600},
d: "javscript"
}
document.write(obj.a);
document.write(obj.b[0]);
document.write(obj.b[1]);
document.write(obj.c.x);
document.write(obj.c.y);
document.write(obj.d);
결과 :
100300400500600javascript
📍 객체 7
키의 값만 배열해 출력이 가능합니다.
let a = 200;
let b = 300;
let c = "javascript";
let obj = {a, b, c};
document.write(a);
document.write(b);
document.write(c);
결과:
200300javascript
📍 객체 8
객체 안에는 함수인 실행문이 들어갈 수 있는데, 실행문을 실행시켜줄 때 쓰는 방법입니다.
const obj = {
a : 200,
b : [300, 400],
c : "javascript",
d : function(){
document.write("javascript가 실행되었습니다.");
},
e : function(){
document.write(obj.c + "가 실행되었습니다."); //변수의 키 값(혹은 변수)을 적고 + 를 쓰면 값이 출력된다.
},
f : function(){
document.write(this.c + "가 실행되었습니다."); //this는 자기 자신을 표현합니다.
}
}
document.write(obj.a);
document.write("<br>");
document.write(obj.b[0]);
document.write("<br>");
document.write(obj.b[1]);
document.write("<br>");
// console.log(obj.b[2]); //undefined
document.write(obj.c);
document.write("<br>");
// console.log(obj.d); //(X)
obj.d();
document.write("<br>");
obj.e();
document.write("<br>");
obj.f();
결과 :
200
300
400
javascript
javascript가 실행되었습니다.
javascript가 실행되었습니다.
javascript가 실행되었습니다.
✨ 감사합니다.
'javascript' 카테고리의 다른 글
데이터 제어문 완벽 정리 (11) | 2023.03.01 |
---|---|
자바스크립트 함수의 종류 마스터 하기 (10) | 2023.02.27 |
자바스크립트 활용 문제 풀어보기 (14) | 2023.02.26 |
자바스크립트 조건문 마스터 하기 (14) | 2023.02.26 |
자바스크립트 반복문 총정리 / 짝수 홀수 구별하는 프로그램 만들기 (17) | 2023.02.23 |